Post Syndicated from Pat Patterson original https://www.backblaze.com/blog/exploring-aws-lite-a-community-driven-javascript-sdk-for-aws/
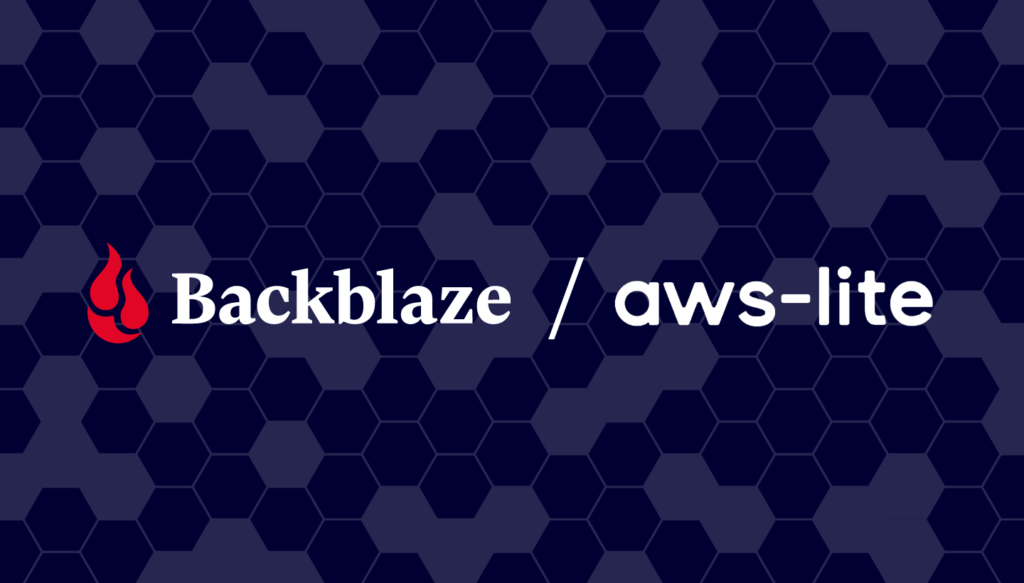
One of the benefits of the Backblaze B2 Storage Cloud having an S3 compatible API is that developers can take advantage of the wide range of Amazon Web Services SDKs when building their apps. The AWS team has released over a dozen SDKs covering a broad range of programming languages, including Java, Python, and JavaScript, and the latter supports both frontend (browser) and backend (Node.js) applications.
With all of this tooling available, you might be surprised to discover aws-lite. In the words of its creators, it is “a simple, extremely fast, extensible Node.js client for interacting with AWS services.” After meeting Brian LeRoux, cofounder and chief technology officer (CTO) of Begin, the company that created the aws-lite project, at the AWS re:Invent conference last year, I decided to give aws-lite a try and share the experience. Read on for the learnings I discovered along the way.
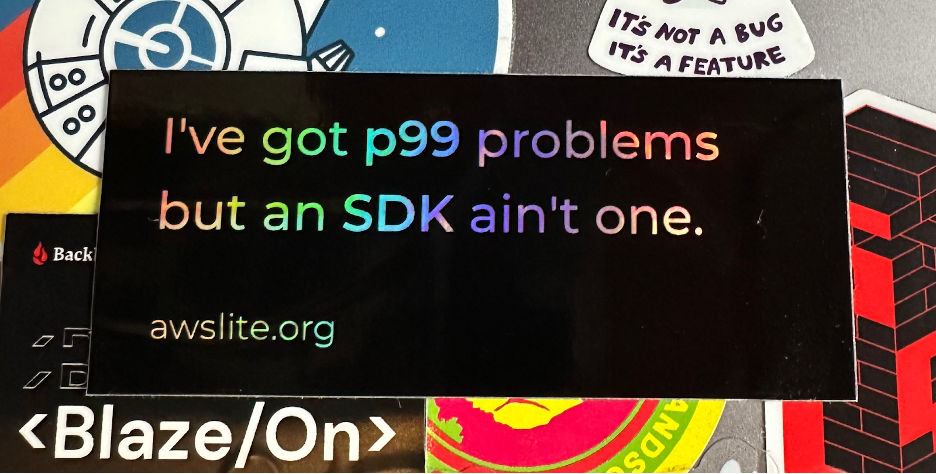
Why Not Just Use the AWS SDK for JavaScript?
The AWS SDK has been through a few iterations. The initial release, way back in May 2013, focused on Node.js, while version 2, released in June 2014, added support for JavaScript running on a web page. We had to wait until December 2020 for the next major revision of the SDK, with version 3 adding TypeScript support and switching to an all-new modular architecture.
However, not all developers saw version 3 as an improvement. Let’s look at a simple example of the evolution of the SDK. The simplest operation you can perform against an S3 compatible cloud object store, such as Backblaze B2, is to list the buckets in an account. Here’s how you would do that in the AWS SDK for JavaScript v2:
var AWS = require('aws-sdk'); var client = new AWS.S3({ region: 'us-west-004', endpoint: 's3.us-west-004.backblazeb2.com' }); client.listBuckets(function (err, data) { if (err) { console.log("Error", err); } else { console.log("Success", data.Buckets); } });
Looking back from 2023, passing a callback function to the listBuckets()
method looks quite archaic! Version 2.3.0 of the SDK, released in 2016, added support for JavaScript promises, and, since async/await arrived in JavaScript in 2017, today we can write the above example a little more clearly and concisely:
const AWS = require('aws-sdk'); const client = new AWS.S3({ region: 'us-west-004', endpoint: 's3.us-west-004.backblazeb2.com' }); try { const data = await client.listBuckets().promise(); console.log("Success", data.Buckets); } catch (err) { console.log("Error", err); }
One major drawback with version 2 of the AWS SDK for JavaScript is that it is a single, monolithic, JavaScript module. The most recent version, 2.1539.0, weighs in at 92.9MB of code and resources. Even the most minimal app using the SDK has to include all that, plus another couple of MB of dependencies, causing performance issues in resource-constrained environments such as internet of things (IoT) devices, or browsers on low-end mobile devices.
Version 3 of the AWS SDK for JavaScript aimed to fix this, taking a modular approach. Rather than a single JavaScript module there are now over 300 packages published under the @aws-sdk/
scope on NPM. Now, rather than the entire SDK, an app using S3 need only install @aws-sdk/client-s3
, which, with its dependencies, adds up to just 20MB.
So, What’s the Problem With AWS SDK for JavaScript v3?
One issue is that, to fully take advantage of modularization, you must adopt an unfamiliar coding style, creating a command object and passing it to the client’s send()
method. Here is the “new way” of listing buckets:
const { S3Client, ListBucketsCommand } = require("@aws-sdk/client-s3"); // Since v3.378, S3Client can read region and endpoint, as well as // credentials, from configuration, so no need to pass any arguments const client = new S3Client(); try { // Inexplicably, you must pass an empty object to // ListBucketsCommand() to avoid the SDK throwing an error const data = await client.send(new ListBucketsCommand({})); console.log("Success", data.Buckets); } catch (err) { console.log("Error", err); }
The second issue is that, to help manage the complexity of keeping the SDK packages in sync with the 200+ services and their APIs, AWS now generates the SDK code from the API specifications. The problem with generated code is that, as the aws-lite home page says, it can result in “large dependencies, poor performance, awkward semantics, difficult to understand documentation, and errors without usable stack traces.”
A couple of these effects are evident even in the short code sample above. The underlying ListBuckets
API call does not accept any parameters, so you might expect to be able to call the ListBucketsCommand
constructor without any arguments. In fact, you have to supply an empty object, otherwise the SDK throws an error. Digging into the error reveals that a module named middleware-sdk-s3
is validating that, if the object passed to the constructor has a Bucket property, it is a valid bucket name. This is a bit odd since, as I mentioned above, ListBuckets
doesn’t take any parameters, let alone a bucket name. The documentation for ListBucketsCommand
contains two code samples, one with the empty object, one without. (I filed an issue for the AWS team to fix this.)
“Okay,” you might be thinking, “I’ll just carry on using v2.” After all, the AWS team is still releasing regular updates, right? Not so fast! When you run the v2 code above, you’ll see the following warning before the list of buckets:
(node:35814) NOTE: We are formalizing our plans to enter AWS SDK for JavaScript (v2) into maintenance mode in 2023. Please migrate your code to use AWS SDK for JavaScript (v3). For more information, check the migration guide at https://a.co/7PzMCcy
At some (as yet unspecified) time in the future, v2 of the SDK will enter maintenance mode, during which, according to the AWS SDKs and Tools maintenance policy, “AWS limits SDK releases to address critical bug fixes and security issues only.” Sometime after that, v2 will reach the end of support, and it will no longer receive any updates or releases.
Getting Started With aws-lite
Faced with a forced migration to what they judged to be an inferior SDK, Brian’s team got to work on aws-lite, posting the initial code to the aws-lite GitHub repository in September last year, under the Apache 2.0 open source license. At present the project comprises a core client and 13 plugins covering a range of AWS services including S3, Lambda, and DynamoDB.
Following the instructions on the aws-lite site, I installed the client module and the S3 plugin, and implemented the ListBuckets
sample:
import awsLite from '@aws-lite/client'; const aws = await awsLite(); try { const data = await aws.S3.ListBuckets(); console.log("Success", data.Buckets); } catch (err) { console.log("Error", err); }
For me, this combines the best of both worlds—concise code, like AWS SDK v2, and full support for modern JavaScript features, like v3. Best of all, the aws-lite client, S3 plugin, and their dependencies occupy just 284KB of disk space, which is less than 2% of the modular AWS SDK’s 20MB, and less than 0.5% of the monolith’s 92.9MB!
Caveat Developer!
(Not to kill the punchline here, but for those of you who might not have studied Latin or law, this is a play on the phrase, “caveat emptor”, meaning “buyer beware”.)
I have to mention, at this point, that aws-lite is still very much under construction. Only a small fraction of AWS services are covered by plugins, although it is possible (with a little extra code) to use the client to call services without a plugin. Also, not all operations are covered by the plugins that do exist. For example, at present, the S3 plugin supports 10 of the most frequently used S3 operations, such as PutObject
, GetObject
, and ListObjectsV2
, leaving the remaining 89 operations TBD.
That said, it’s straightforward to add more operations and services, and the aws-lite team welcomes pull requests. We’re big believers in being active participants in the open source community, and I’ve already contributed the ListBuckets
operation, a fix for HeadObject
, and I’m working on adding tests for the S3 plugin using a mock S3 server. If you’re a JavaScript developer working with cloud services, this is a great opportunity to contribute to an open source project that promises to make your coding life better!
The post Exploring aws-lite, a Community-Driven JavaScript SDK for AWS appeared first on Backblaze Blog | Cloud Storage & Cloud Backup.